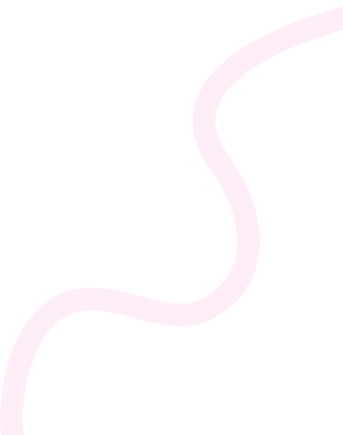
- Home
- 200 Laravel Interview Questions and Answers 2024
- Explain how to use the `contains` method in Laravel collections.
Explain how to use the `contains` method in Laravel collections.
Answer: In Laravel, the `contains` method is used to determine if a collection contains a given item. You can use it in several ways:
1. By value: Checks if a specific value exists in the collection.
“`php
$collection = collect([1, 2, 3, 4]);
$contains = $collection->contains(2); // Returns true
“`
2. By key-value pair: Checks if a collection of key-value pairs contains a specified key-value combination.
“`php
$collection = collect([‘name’ => ‘John’, ‘age’ => 25]);
$contains = $collection->contains(‘name’, ‘John’); // Returns true
“`
3. Using a callback: Pass a callback function to determine if any item meets a specified condition.
“`php
$collection = collect([1, 2, 3, 4]);
$contains = $collection->contains(function ($value) {
return $value > 2; // Returns true
});
“`
Use `contains` when you need to check for item existence efficiently within a Laravel collection.
Related Questions & Topics
-
- 1 min read
What are Doctrine Lifecycle Callbacks?
-
- 1 min read
How do you use Phalcon’s dependency injection for better code organization?
-
- 1 min read
What is Phalcon’s approach to handling application exceptions?
-
- 1 min read
How do you use Yii’s Validator class for custom validation logic?
-
- 1 min read
How do you use Joomla’s JModel class for database interactions?
-
- 1 min read
What are the best practices for managing passwords in Magento?
-
- 1 min read
What is the role of Magento’s static-content:deploy command?
-
- 1 min read
How do you manage search functionality in Concrete?
-
- 1 min read
Explain how Magento’s fallback mechanism works for themes.
-
- 1 min read
How do you schedule a job in Concrete?
-
- 1 min read
Explain how to use Magento’s integration testing framework.
-
- 1 min read
How do you handle content delivery for high-traffic Ghost sites?
-
- 1 min read
What is the purpose of Ghost’s API, and how is it used?
-
- 1 min read
What are the best practices for CMS backup and disaster recovery?
-
- 1 min read
How do you handle content repurposing and updating in a CMS?
-
- 1 min read
How do you use CDN for improving Ghost’s performance?
-
- 1 min read
How do you create a custom social media block in Concrete?
-
- 1 min read
What is the role of the PrestaShop Front Controller?
-
- 1 min read
What is the process for integrating Slim Framework with an authentication provider?
-
- 1 min read
How do you pass data to a Blade view?
-
- 1 min read
Explain how to manage multisite installations with Composer in Drupal.
-
- 1 min read
How do you define validation rules in FuelPHP ORM models?
-
- 1 min read
How do you handle customer data protection in Magento?
-
- 1 min read
Can you explain the process of creating and managing content types in a CMS?
-
- 1 min read
What are Phalcon’s built-in validation methods?
-
- 1 min read
How do you handle pagination in Magento’s REST API?
-
- 1 min read
What are PrestaShop’s options for handling product variations?
-
- 1 min read
What is the purpose of catalog price rules in Magento?
-
- 1 min read
What is a cell in CakePHP, and when would you use it?
-
- 1 min read
How do you manage custom form fields and validation in SilverStripe?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework