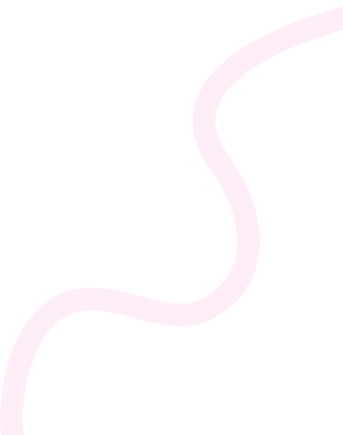
- Home
- 199 SlimInterview Questions and Answers 2024
- How can you implement dependency injection in Slim Framework?
How can you implement dependency injection in Slim Framework?
To implement Dependency Injection (DI) in the Slim Framework, you can use its built-in container (based on PSR-11 standard). Here’s a minimal guide to implementing DI in Slim:
Steps to Implement Dependency Injection in Slim Framework:
Set Up a Dependency Container: Slim uses a PSR-11 compliant container (like PHP-DI or Pimple) to manage dependencies. You can define services in the container.
Example
<?php
use Psr\Container\ContainerInterface;
use Slim\Factory\AppFactory;
// Create App with a container
$container = new \DI\Container(); // PHP-DI container
AppFactory::setContainer($container);
$app = AppFactory::create();
?>
- Register Dependencies in the Container:
logger
service or any class. Example
<?php
// Register a service in the container
$container->set('logger', function (ContainerInterface $c) {
$logger = new \Monolog\Logger('app');
$logger->pushHandler(new \Monolog\Handler\StreamHandler('path/to/logfile.log'));
return $logger;
});
?>
- Use Dependency Injection in Routes:
Example
<?php
$app->get('/log', function ($request, $response, $args) {
// Access the logger service from the container
$logger = $this->get('logger');
$logger->info('Logging info from the route');
$response->getBody()->write("Logged successfully!");
return $response;
});
?>
- Inject Dependencies into Controllers or Classes: You can also inject dependencies into custom classes (like controllers) by accessing the container in your class constructor.
Example
<?php
class MyController
{
protected $logger;
// Inject the logger dependency
public function __construct(ContainerInterface $container)
{
$this->logger = $container->get('logger');
}
public function logSomething($request, $response)
{
$this->logger->info('Logged from controller');
$response->getBody()->write("Logged from controller");
return $response;
}
}
// Add route with injected controller
$app->get('/controller-log', [MyController::class, 'logSomething']);
?>
- Run the Application:
Example
<?php
$app->run();
?>
Related Questions & Topics
Other Interview Question Answers
-
- 1 min read
How does Phalcon handle HTTP request and response objects?
-
- 1 min read
How do you create a custom sitemap.xml in Concrete?
-
- 1 min read
How do you implement OAuth in Laravel APIs?
-
- 1 min read
Describe the Drupal taxonomy system.
-
- 1 min read
How do you use Slim Framework with a web services integration?
-
- 1 min read
How do you set up a Zend Framework project from scratch?
-
- 1 min read
How can you define custom routes in Yii?
-
- 1 min read
How do you implement session encryption in FuelPHP?
-
- 1 min read
What is eager loading in Laravel, and why is it important?
-
- 1 min read
What is a pivot table in Laravel, and how do you use it?
-
- 1 min read
How do you track changes and revisions in Drupal content?
-
- 1 min read
Explain the architecture of Drupal.
-
- 1 min read
What are PrestaShop’s features for managing taxes?
-
- 1 min read
How do you use register_post_type() for custom post types?
-
- 1 min read
How do you configure Magento for high availability?
-
- 1 min read
What tools and techniques do you use for CMS project management?
-
- 1 min read
How do you create a custom podcast block in Concrete?
-
- 1 min read
How do you configure Ghost for different environments (development, production)?
-
- 1 min read
How do you test custom features or functionalities in a CMS?
-
- 1 min read
How can you implement authentication in Zend Framework?
-
- 1 min read
How do you handle image optimization in Magento?
-
- 1 min read
How do you manage redirects in Concrete?
-
- 1 min read
How do you handle third-party API integrations in Yii?
-
- 1 min read
Explain the process of developing and testing PrestaShop modules.
-
- 1 min read
Explain the role of the DataExtension class in extending SilverStripe’s functionality.
-
- 1 min read
How do you manage large product catalogs in PrestaShop?
-
- 1 min read
What are the steps to integrate Joomla with a CRM system?
-
- 1 min read
What is the Content Moderation module in Drupal?
-
- 1 min read
How do you implement Joomla with a secure backup strategy?
-
- 1 min read
What are some common usability issues in CMS platforms, and how do you address them?
Other Interview Question Answers
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework