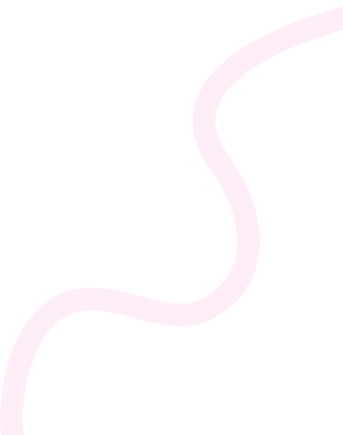
- Home
- 199 Zend Framework Interview Questions and Answers 2024
- How can you use Zend_Db_Adapter_Pdo_Mysql for MySQL databases?
How can you use Zend_Db_Adapter_Pdo_Mysql for MySQL databases?
Using Zend_Db_Adapter_Pdo_Mysql
in Zend Framework allows you to interact with MySQL databases through a PDO (PHP Data Objects) adapter. This adapter provides a consistent interface for database operations while leveraging the PDO extension’s features. Here’s a step-by-step guide to set up and use Zend_Db_Adapter_Pdo_Mysql
for MySQL databases.
Steps to Use Zend_Db_Adapter_Pdo_Mysql for MySQL Databases
Step 1: Install Zend Framework
Ensure you have Zend Framework installed in your project. If you haven’t installed it yet, you can use Composer:
Example
composer require zendframework/zend-db
Step 2: Configure the Database Adapter
You need to configure the Zend_Db_Adapter_Pdo_Mysql
instance with your database connection details, such as the hostname, database name, username, and password.
Example: Configuration
You can set up the adapter directly in your application configuration or create a factory class to instantiate the adapter.
Example
<?php
use Zend\Db\Adapter\Adapter;
use Zend\Db\Adapter\AdapterInterface;
$dbConfig = [
'driver' => 'Pdo_Mysql',
'database' => 'your_database_name',
'username' => 'your_username',
'password' => 'your_password',
'hostname' => 'localhost', // or your MySQL server IP
];
$adapter = new Adapter($dbConfig);
?>
Step 3: Basic CRUD Operations
Once the adapter is configured, you can perform basic CRUD (Create, Read, Update, Delete) operations using it.
Example: Create
Example
<?php
$sql = "INSERT INTO users (name, email) VALUES (:name, :email)";
$statement = $adapter->createStatement($sql);
$statement->setParameter(':name', 'John Doe');
$statement->setParameter(':email', 'john@example.com');
$result = $statement->execute();
?>
Example: Read
Example
<?php
$sql = "SELECT * FROM users WHERE email = :email";
$statement = $adapter->createStatement($sql);
$statement->setParameter(':email', 'john@example.com');
$result = $statement->execute();
if ($result->count()) {
foreach ($result as $row) {
echo $row['name'] . " - " . $row['email'];
}
}
?>
Example: Update
Example
<?php
$sql = "UPDATE users SET name = :name WHERE email = :email";
$statement = $adapter->createStatement($sql);
$statement->setParameter(':name', 'Jane Doe');
$statement->setParameter(':email', 'john@example.com');
$result = $statement->execute();
?>
Example: Delete
Example
<?php
$sql = "DELETE FROM users WHERE email = :email";
$statement = $adapter->createStatement($sql);
$statement->setParameter(':email', 'john@example.com');
$result = $statement->execute();
?>
Step 4: Handle Transactions (Optional)
You can also handle transactions with Zend_Db_Adapter_Pdo_Mysql
to ensure data integrity.
Example
<?php
$adapter->getDriver()->getConnection()->beginTransaction();
try {
// Perform multiple operations here
$adapter->getDriver()->getConnection()->commit();
} catch (\Exception $e) {
$adapter->getDriver()->getConnection()->rollback();
throw $e; // Handle the exception as needed
}
?>
Related Questions & Topics
-
- 1 min read
What is a reverse proxy, and how can it be used with Drupal?
-
- 1 min read
How do you implement custom form validation rules in SilverStripe?
-
- 1 min read
How do you handle order processing and fulfillment in PrestaShop?
-
- 1 min read
How do you troubleshoot issues with product imports in PrestaShop?
-
- 1 min read
How do you manage timezones in CakePHP?
-
- 1 min read
How do you implement multilingual support in Drupal?
-
- 1 min read
How does FuelPHP handle input filtering for security?
-
- 1 min read
How do you handle and prevent unauthorized access to CMS admin areas?
-
- 1 min read
How do you troubleshoot common deployment issues in Magento?
-
- 1 min read
How do you use TYPO’s Fluid templates to build responsive layouts?
-
- 1 min read
How do you use Phalcon’s PhalconMvcModelQueryLang for dynamic queries?
-
- 1 min read
How do you create and use custom SilverStripe modules?
-
- 1 min read
How do you install Ghost on a server?
-
- 1 min read
How do you implement file caching in Phalcon?
-
- 1 min read
How do you secure the Magento Admin panel?
-
- 1 min read
How do you implement API endpoints using Zend Framework?
-
- 1 min read
What is Yii’s “Gii Generator” and how does it simplify development?
-
- 1 min read
What are “jobs” in Concrete, and how are they used?
-
- 1 min read
How do you handle backups and disaster recovery for Ghost?
-
- 1 min read
What is the MVC architecture in CodeIgniter?
-
- 1 min read
Describe the folder structure of a Ghost theme.
-
- 1 min read
What is the purpose of the `Model` class in FuelPHP?
-
- 1 min read
What are Symfony’s best practices for API versioning?
-
- 1 min read
How do you implement custom user roles in Ghost?
-
- 1 min read
What is the Entity API in Drupal?
-
- 1 min read
What are the steps involved in deploying a Symfony application?
-
- 1 min read
What are Phalcon’s tools for managing application security?
-
- 1 min read
What are some common issues with WooCommerce and how do you resolve them?
-
- 1 min read
How do you handle database migrations in Magento?
-
- 1 min read
What are WordPress hooks and how are they used in plugins?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework