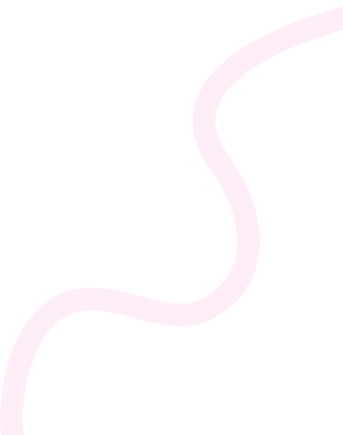
- Home
- Fuel PHP Interview Questions and Answers 2024
- How do you create custom classes in FuelPHP?
How do you create custom classes in FuelPHP?
To create custom classes in FuelPHP, follow these steps with practical examples for clarity:
1. Create the Class File
Place your custom class file in the classes/
directory, adhering to the PSR-4 naming convention. This means the file structure should mirror the class namespace. For example, let’s create a file called MyClass.php
in the classes/
directory.
Example
fuel/app/classes/MyNamespace/MyClass.php
2. Define the Class
Within MyClass.php
, define your class. The namespace should match the folder structure. Here’s an example:
Example
<?php
namespace MyNamespace;
class MyClass {
// Example of a method that could process some input
public function myMethod($input) {
return "Hello, " . $input;
}
}
?>
In this case, MyClass
has a simple method myMethod
that takes an input and returns a greeting message.
3. Autoload the Class
FuelPHP uses its autoloader to load any class in the classes/
directory automatically, as long as the file and namespace follow the correct conventions. There’s no need to manually include or require the file.
FuelPHP takes care of autoloading, so the following step isn’t required in most cases, but for clarity:
- Just ensure the file is placed correctly under the
classes/
folder.
4. Use the Class
You can now use your custom class in any part of your application, such as in controllers or models. First, make sure to import the class using the correct namespace with use
.
Here’s how you could use the class in a controller:
Example
<?php
use MyNamespace\MyClass;
class Controller_Home extends Controller {
public function action_index() {
// Create an instance of MyClass
$instance = new MyClass();
// Call the method and display the result
$message = $instance->myMethod('World');
// Output the message (for example purposes)
return Response::forge($message);
}
}
?>
Example Output:
In the browser, navigating to the route handled by Controller_Home
would result in:
Example
Hello, World
Important Notes:
- PSR-4 Compatibility: Ensure that the folder structure mirrors the namespace.
MyNamespace\MyClass
should reside infuel/app/classes/MyNamespace/MyClass.php
.
- FuelPHP Coding Standards: Stick to FuelPHP’s conventions for naming and structuring files, which helps with maintainability and avoids issues with the autoloader.
By following these steps, you can create and use custom classes seamlessly in your FuelPHP application!
Related Questions & Topics
-
- 1 min read
How do you manage relationships between DataObject classes in SilverStripe?
-
- 1 min read
How do you use Phalcon’s built-in methods for form processing?
-
- 1 min read
How do you identify and fix slow queries in Magento?
-
- 1 min read
How do you improve the user experience on a WordPress site?
-
- 1 min read
How do you handle image uploads and media management in SilverStripe?
-
- 1 min read
How do you integrate PrestaShop with social media platforms?
-
- 1 min read
What is the use of wp_enqueue_scripts hook in a plugin?
-
- 1 min read
How do you integrate a custom messaging system in Concrete?
-
- 1 min read
Explain how to use Memcached with Drupal.
-
- 1 min read
What are soft deletes, and how does FuelPHP ORM handle them?
-
- 1 min read
What are TYPO’s best practices for backend user management?
-
- 1 min read
What are the key features of SilverStripe’s ORM system?
-
- 1 min read
What are the best practices for data privacy and compliance in analytics?
-
- 1 min read
What is the purpose of the wp_nonce_field() function?
-
- 1 min read
How do you use the oil command for testing in FuelPHP?
-
- 1 min read
How do you handle real-time data synchronization between Drupal and external systems?
-
- 1 min read
How do you create a custom form in Joomla using JForm?
-
- 1 min read
What are the default database settings in Ghost, and how can they be changed?
-
- 1 min read
What is the significance of PCI compliance in Magento?
-
- 1 min read
How do you handle API versioning in Drupal?
-
- 1 min read
How do you implement custom routes in Ghost?
-
- 1 min read
Explain the concept of “dependency injection” in Yii.
-
- 1 min read
How do you manage widget visibility on different pages?
-
- 1 min read
How do you add a custom job to the Concrete task scheduler?
-
- 1 min read
How do you implement logging in Slim Framework?
-
- 1 min read
How do you use SilverStripe’s GridField for data management?
-
- 1 min read
How do you handle form validation in FuelPHP?
-
- 1 min read
How do you enable and configure hooks in CodeIgniter?
-
- 1 min read
What are Symfony’s best practices for creating reusable components?
-
- 1 min read
Explain the use of wp_head() and wp_footer() hooks in a theme.
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework