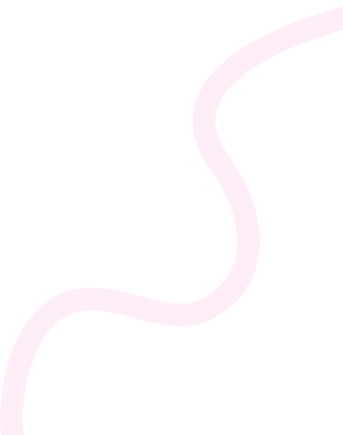
- Home
- 200 Laravel Interview Questions and Answers 2024
- How do you create custom gates in Laravel?
How do you create custom gates in Laravel?
Answer: In Laravel, you can create custom gates by using the `Gate` facade. Here’s how:
1. Define your gate: In the `boot` method of a service provider (usually `AuthServiceProvider`), use the `Gate::define` method. For example:
“`php
use IlluminateSupportFacadesGate;
public function boot()
{
Gate::define(‘view-dashboard’, function ($user) {
return $user->isAdmin(); // Customize this logic as needed
});
}
“`
2. Authorize Actions: Use the `can` method in your controllers or views to check permissions.
“`php
if (Gate::allows(‘view-dashboard’)) {
// The user can view the dashboard
}
“`
3. Blade Syntax: You can also use Blade directives to check gates directly in your views:
“`blade
@can(‘view-dashboard’)
You can view the dashboard!
@endcan
“`
By following these steps, you can create and manage custom gates in your Laravel application.
Related Questions & Topics
-
- 1 min read
How do you implement custom middleware in Slim Framework?
-
- 1 min read
How do you set up a new SilverStripe project from scratch?
-
- 1 min read
What are Symfony’s best practices for testing?
-
- 1 min read
Describe the process of implementing a custom middleware stack in Slim Framework.
-
- 1 min read
What is the Migrate module in Drupal?
-
- 1 min read
Explain how to use the Locale module for translation management in Drupal.
-
- 1 min read
What is the role of the “Page Controller” in Concrete?
-
- 1 min read
How do you test events in Laravel?
-
- 1 min read
What is the purpose of wp_register_script() and wp_enqueue_script()?
-
- 1 min read
Describe how to manage user roles and permissions in Ghost.
-
- 1 min read
How do you secure a CakePHP application?
-
- 1 min read
How do you use Zend_Validate_Between for range validation?
-
- 1 min read
What are PrestaShop’s built-in reporting features?
-
- 1 min read
How do you perform security audits on a CMS?
-
- 1 min read
How do you install CodeIgniter?
-
- 1 min read
What are Joomla extensions, and how are they categorized?
-
- 1 min read
What is the purpose of the Joomla Global Configuration settings?
-
- 1 min read
What is the role of the Form class in SilverStripe, and how is it used?
-
- 1 min read
How do you extend the functionality of SilverStripe’s default admin interface?
-
- 1 min read
How do you define a route with optional parameters in Laravel?
-
- 1 min read
What are some best practices for securing API endpoints?
-
- 1 min read
How do you perform regression testing for WordPress updates?
-
- 1 min read
How do you handle and manage risks and issues in CMS projects?
-
- 1 min read
Explain how to use the Query Monitor plugin.
-
- 1 min read
How do you secure sensitive data using Yii’s encryption methods?
-
- 1 min read
What is Joomla’s Template Style feature, and how is it used?
-
- 1 min read
How do you monitor Joomla for security vulnerabilities?
-
- 1 min read
What are TYPO’s built-in methods for handling SEO?
-
- 1 min read
How do you create a custom CMS block in Magento?
-
- 1 min read
What are Joomla’s built-in security features?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework