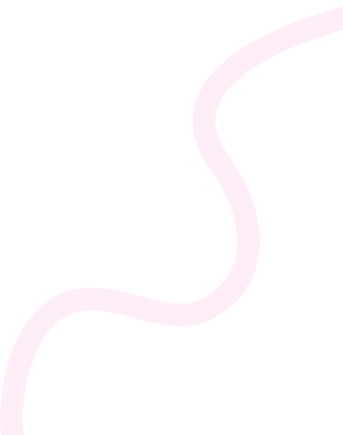
- Home
- 199 Zend Framework Interview Questions and Answers 2024
- How do you implement a custom validator in Zend Framework?
How do you implement a custom validator in Zend Framework?
Answer: To implement a custom validator in Zend Framework, follow these steps:
1. Create the Validator Class: Extend `ZendValidatorAbstractValidator` and implement the necessary methods, such as `isValid()`.
2. Define Error Messages: Use the `setMessage()` method to define validation error messages.
3. Register the Validator: If needed, register your custom validator using `ZendValidatorAbstractValidator::setTranslationTextDomain()`.
4. Use the Validator: In your form or model, use your custom validator by creating an instance and calling it within the validation process.
5. Integrate with Forms: Add your custom validator to the form’s input filter.
Example code for a simple custom validator:
“`php
namespace MyValidator;
use ZendValidatorAbstractValidator;
class MyCustomValidator extends AbstractValidator
{
const NOT_VALID = ‘notValid’;
protected $messageTemplates = [
self::NOT_VALID => ‘Value is not valid’,
];
public function isValid($value)
{
$this->setValue($value);
// Add your validation logic here
if ($value !== ‘expectedValue’) {
$this->error(self::NOT_VALID);
return false;
}
return true;
}
}
“`
Use it in a form:
“`php
$form->getInputFilter()->get(‘fieldName’)->setValidator(new MyValidatorMyCustomValidator());
“`
This approach allows you to create robust, reusable validation logic in your Zend Framework applications.
Related Questions & Topics
-
- 1 min read
What is the purpose of the Config Split module in Drupal?
-
- 1 min read
What are sparks in CodeIgniter?
-
- 1 min read
How do you handle job timeouts in Laravel?
-
- 1 min read
How does Phalcon support web application security features?
-
- 1 min read
What are the steps for implementing GDPR features in PrestaShop?
-
- 1 min read
How do you implement custom fonts in a Magento theme?
-
- 1 min read
How do you use Phalcon’s PhalconCacheBackendRedis class?
-
- 1 min read
How do you add Google Tag Manager to a Concrete site?
-
- 1 min read
How do you create a new controller in CakePHP?
-
- 1 min read
How do you create custom discounts and promotions in PrestaShop?
-
- 1 min read
How do you create and use Phalcon’s CLI commands?
-
- 1 min read
How do you perform database migrations in CakePHP?
-
- 1 min read
How do you handle PHP errors in WordPress?
-
- 1 min read
How do you create a new database table in Magento?
-
- 1 min read
How do you create a Blade component in Laravel?
-
- 1 min read
How do you configure Zend Framework for different environments (development, production)?
-
- 1 min read
What is a TYPO Page Template?
-
- 1 min read
What are the best practices for releasing and distributing Magento extensions?
-
- 1 min read
How do you handle API rate limiting in Drupal?
-
- 1 min read
How do you work with Concrete’s permission model programmatically?
-
- 1 min read
What are some strategies for managing and updating large volumes of content?
-
- 1 min read
What is a hook in CodeIgniter?
-
- 1 min read
What are presenters in FuelPHP, and when would you use them?
-
- 1 min read
How do you create a custom widget for SilverStripe’s admin interface?
-
- 1 min read
How do you create a custom integration with an external API in Concrete?
-
- 1 min read
How do you manage email campaigns and newsletters in PrestaShop?
-
- 1 min read
How do you create custom controllers in Yii?
-
- 1 min read
How does Magento handle multi-store setups?
-
- 1 min read
How do you create a blog in Concrete?
-
- 1 min read
Explain the concept of Symfony services.
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework