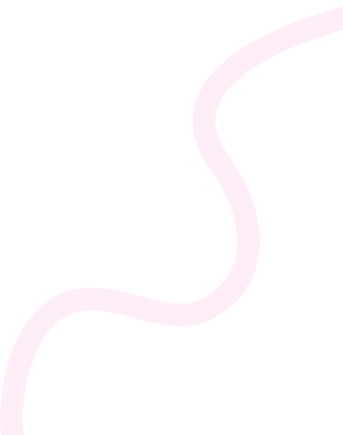
- Home
- Fuel PHP Interview Questions and Answers 2024
- How do you protect routes in FuelPHP based on user roles?
How do you protect routes in FuelPHP based on user roles?
To protect routes in FuelPHP based on user roles, you can use the Auth
package, which supports role-based access control (RBAC). Here’s how you can restrict access to routes based on user roles in minimal steps:
Step 1: Ensure Auth
Package is Loaded
Ensure that the auth
package is loaded in your configuration file
Example
<?php
'always_load' => array(
'packages' => array(
'auth',
),
),
?>
Step 2: Define User Roles
Ensure that user roles are set up in simpleauth.php
. By default, the groups
array defines user roles:
Example
<?php
// In fuel/app/config/simpleauth.php
'groups' => array(
-1 => array('name' => 'Banned', 'roles' => array('banned')),
0 => array('name' => 'Guests', 'roles' => array('guest')),
1 => array('name' => 'Users', 'roles' => array('user')),
100 => array('name' => 'Administrators', 'roles' => array('admin')),
),
?>
Step 3: Protect Routes in the Controller
You can use the Auth::member()
method in the controller’s before()
method to restrict access based on user roles. For example, to only allow administrators access to certain actions:
Example
<?php
class Controller_Admin extends Controller
{
public function before()
{
parent::before();
// Check if the user is logged in
if (!Auth::check()) {
// Redirect to login page
Response::redirect('login');
}
// Check if the user is an administrator (group 100)
if (!Auth::member(100)) {
// Redirect to a "403 Forbidden" page if not an admin
Response::redirect('403');
}
}
public function action_dashboard()
{
// Admin dashboard code here
return 'Admin Dashboard';
}
}
?>
Step 4: Protect Specific Methods
You can also protect specific controller methods directly:
Example
<?php
public function action_edit()
{
// Check if the user belongs to the moderator group (group 50)
if (!Auth::member(50)) {
// Redirect or show an error message
Response::redirect('403');
}
// Code for editing content
}
?>
Step 5: Redirect Unauthorized Users to 403 Page
Create a simple 403 forbidden page:
Example
<?php
class Controller_403 extends Controller
{
public function action_index()
{
return 'Access denied. You do not have permission to view this page.';
}
}
?>
Related Questions & Topics
Other Interview Question Answers
-
- 1 min read
What is the purpose of register_sidebar()?
-
- 1 min read
How do you set session cookies in FuelPHP?
-
- 1 min read
Describe how to use Ghost’s membership features for user engagement.
-
- 1 min read
How do you create a custom location block in Concrete?
-
- 1 min read
How do you create a TYPO extension?
-
- 1 min read
How do you handle image optimization in Magento?
-
- 1 min read
How do you handle custom URL routing in SilverStripe?
-
- 1 min read
What are the best practices for CMS backup and disaster recovery?
-
- 1 min read
How do you install a new module in Drupal?
-
- 1 min read
Explain how migrations work in FuelPHP.
-
- 1 min read
How do you handle file and image uploads in Yii?
-
- 1 min read
Explain the process of creating a multilingual site in Joomla.
-
- 1 min read
How do you create and use custom SilverStripe modules?
-
- 1 min read
What is the process for creating a custom plugin or extension for a CMS?
-
- 1 min read
How do you properly use the register_activation_hook() function in a plugin?
-
- 1 min read
What are TYPO’s methods for handling file uploads and storage?
-
- 1 min read
How does Zend Framework handle HTTP headers?
-
- 1 min read
What impact does artificial intelligence have on CMS platforms?
-
- 1 min read
How do you develop a content strategy for a CMS-based site?
-
- 1 min read
What is Zend_View_Helper_FormTextarea and how is it used?
-
- 1 min read
How do you integrate Slim Framework with a messaging and notification service?
-
- 1 min read
What are the benefits of using an opcode cache with Symfony?
-
- 1 min read
Explain how to test APIs in Laravel.
-
- 1 min read
What strategies do you use for CMS backup and disaster recovery?
-
- 1 min read
How do you integrate TYPO with a third-party payment gateway?
-
- 1 min read
Describe the purpose and usage of Yii’s “Controller” class.
-
- 1 min read
How do you create and configure custom dashboard widgets in PrestaShop?
-
- 1 min read
How do you use TYPO’s TypoScript to manage site-wide settings?
-
- 1 min read
Explain the concept of Doctrine repositories.
-
- 1 min read
How do you create a custom contact form in Concrete?
Other Interview Question Answers
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework