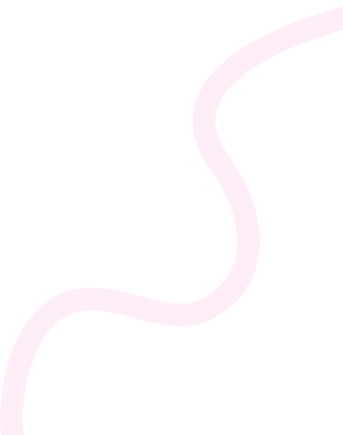
- Home
- 199 SlimInterview Questions and Answers 2024
- Describe how you would implement pagination in Slim Framework.
Describe how you would implement pagination in Slim Framework.
To implement pagination in Slim Framework, you can follow these steps:
Step 1: Set up a GET route for the paginated data
Define a route that accepts page numbers as a query parameter or a route parameter. This route will fetch and return the paginated data.
Example
<?php
use Psr\Http\Message\ResponseInterface as Response;
use Psr\Http\Message\ServerRequestInterface as Request;
use Slim\Factory\AppFactory;
require __DIR__ . '/vendor/autoload.php';
$app = AppFactory::create();
$app->get('/items', function (Request $request, Response $response) {
// Step 2: Fetch the page number from query parameters (or set default to 1)
$queryParams = $request->getQueryParams();
$page = isset($queryParams['page']) ? (int)$queryParams['page'] : 1;
$perPage = 5; // Number of items per page
// Step 3: Simulate a data source (e.g., an array of items)
$items = range(1, 100); // Example data
$totalItems = count($items);
// Step 4: Calculate pagination offsets
$offset = ($page - 1) * $perPage;
$paginatedItems = array_slice($items, $offset, $perPage);
// Step 5: Create a response with the paginated data
$pagination = [
'current_page' => $page,
'per_page' => $perPage,
'total_items' => $totalItems,
'total_pages' => ceil($totalItems / $perPage),
'data' => $paginatedItems
];
$response->getBody()->write(json_encode($pagination));
return $response->withHeader('Content-Type', 'application/json');
});
$app->run();
?>
Step 2: Fetch the page number
In the route, retrieve the page number from the query parameters (e.g., ?page=2
). If no page is specified, default to page 1.
Step 3: Simulate or fetch data
For the purpose of this example, an array of numbers (1 to 100) is used as the data source. In a real application, you’d query a database or external API to fetch the data.
Step 4: Calculate the pagination offsets
Using the page number, calculate the offset for slicing the data and limit the number of items returned based on the perPage
value.
Step 5: Return paginated data
Return the paginated data along with pagination metadata (e.g., current_page
, total_items
, total_pages
, etc.) as a JSON response.
Step 6: Test the Pagination
Start your Slim app and test the pagination by calling the /items?page=1
route with different page numbers.
This simple approach can be expanded to handle larger data sets and more complex data retrieval methods (like using a database).
Related Questions & Topics
-
- 1 min read
How do you implement caching in Zend Framework?
-
- 1 min read
How does Zend Framework handle pagination with Zend_Paginator?
-
- 1 min read
How do you use the WebProfilerBundle in Symfony?
-
- 1 min read
What is the role of Magento’s static-content:deploy command?
-
- 1 min read
How can you handle URL parameters in FuelPHP routing?
-
- 1 min read
What is the difference between GET and POST methods in CodeIgniter?
-
- 1 min read
How do you implement RESTful APIs in FuelPHP?
-
- 1 min read
Explain how to manage users across multiple sites in Drupal.
-
- 1 min read
How do you define a model in FuelPHP?
-
- 1 min read
How do you handle pagination and filtering with the Ghost API?
-
- 1 min read
Describe the use of Zend_Layout in a Zend Framework application.
-
- 1 min read
Explain the use of Symfony’s service container for custom services.
-
- 1 min read
Describe the use of Yii’s “HttpException” class.
-
- 1 min read
How do you secure Joomla’s API keys?
-
- 1 min read
How do you optimize FuelPHP for performance?
-
- 1 min read
What are the security best practices for Joomla?
-
- 1 min read
How do you create a custom admin controller in Magento?
-
- 1 min read
How does SilverStripe handle routing for custom controllers?
-
- 1 min read
What is the PrestaShop cron job system used for?
-
- 1 min read
How do you handle rolling deployments with Symfony?
-
- 1 min read
Describe the TYPO cache layers and their purposes.
-
- 1 min read
Describe the process of configuring SSL for Ghost.
-
- 1 min read
What are the best practices for handling API security in Slim Framework?
-
- 1 min read
How do you use Twig templates in Symfony?
-
- 1 min read
How do you implement accessibility features in a WordPress theme?
-
- 1 min read
How do you perform cross-browser testing on a Magento site?
-
- 1 min read
Can you describe the process of gathering and defining CMS project requirements?
-
- 1 min read
How do you secure Joomla’s email forms from spam?
-
- 1 min read
What is the purpose of the `config.production.json` file in Ghost?
-
- 1 min read
What are SilverStripe’s built-in logging features, and how do you utilize them?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework