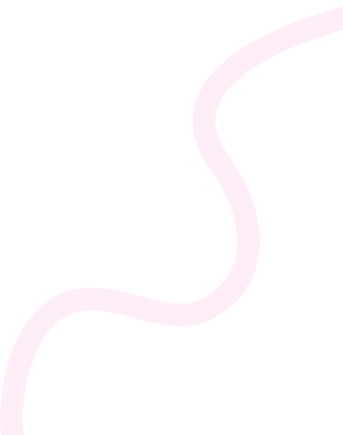
- Home
- Fuel PHP Interview Questions and Answers 2024
- Explain how to use the to_array() method in FuelPHP ORM.
Explain how to use the to_array() method in FuelPHP ORM.
In FuelPHP ORM, the to_array()
method is commonly used to transform an ORM model instance into an associative array. This is particularly useful for API responses, or when data needs to be serialized for JSON output, making the data easier to manage and manipulate in your application.
Practical Examples:
- Basic Usage Example:
Let’s assume you have a model Model_Product
, and you want to fetch a product by its ID and convert it into an array for further processing or sending in a JSON response.
Example
<?php
$product = Model_Product::find($id);
$array = $product->to_array();
?>
In this case, $product->to_array()
will take all the attributes of the Model_Product
instance (like name
, price
, description
, etc.) and convert them into an associative array.
For example, if your Model_Product
has the following attributes:
id
=> 101name
=> ‘FuelPHP Book’price
=> 29.99
The result of to_array()
would look like:
Example
<?php
Array
(
[id] => 101
[name] => FuelPHP Book
[price] => 29.99
)
?>
- Including Related Models:
You can also include related models by passing true
as an argument to to_array()
. This will include any related models (e.g., Category
, Reviews
, or Tags
) in the resulting array.
For example, if a Product
belongs to a Category
and has related Reviews
, you can include these relationships:
Example
<?php
$product = Model_Product::find($id);
$array = $product->to_array(true); // Includes related models
?>
Suppose the related data looks like this:
Category
:Books
Reviews
:[Good read!, Highly recommended]
The resulting array would look like:
Example
<?php
Array
(
[id] => 101
[name] => FuelPHP Book
[price] => 29.99
[category] => Array
(
[id] => 5
[name] => Books
)
[reviews] => Array
(
[0] => Good read!
[1] => Highly recommended
)
)
?>
Use Cases:
API Responses: When creating an API endpoint, you can easily convert the model to an array and then return it as JSON:
Example
<?php
$product = Model_Product::find($id);
return json_encode($product->to_array());
?>
- Templating or Front-End Integration: You can use the associative array to pass data to a template, making it easy to work with in views or for integrating with front-end frameworks.
By using to_array()
, you streamline the conversion of ORM model data into a more flexible format, simplifying data manipulation and presentation across different layers of your application.
Related Questions & Topics
-
- 1 min read
How do you use Zend_Db_Adapter_Pdo_Sqlsrv for SQL Server databases?
-
- 1 min read
Explain the concept of “polymorphic” relationships in SilverStripe and how to implement them.
-
- 1 min read
How do you use Joomla’s JLoader class for class loading?
-
- 1 min read
How can you inject services into a Symfony controller?
-
- 1 min read
What is the “Dashboard” in Concrete, and how do you customize it?
-
- 1 min read
Can you explain how to resolve issues with CMS media management?
-
- 1 min read
How do you connect Ghost with email marketing tools?
-
- 1 min read
How do you implement CAPTCHA in Magento forms?
-
- 1 min read
What is the purpose of the `middleware` method in Laravel controllers for authentication?
-
- 1 min read
How do you define routes in Slim Framework?
-
- 1 min read
What is the purpose of register_deactivation_hook() in a plugin?
-
- 1 min read
How do you sort results using Eloquent?
-
- 1 min read
What are the best practices for integrating third-party services with Ghost?
-
- 1 min read
What is the process for migrating content from one CMS to another?
-
- 1 min read
What are the different stages of a Drupal migration?
-
- 1 min read
What is Phalcon’s PhalconMvcModelBehavior class used for?
-
- 1 min read
What are common performance issues in CMS platforms, and how can they be resolved?
-
- 1 min read
How do you handle broken links in Drupal?
-
- 1 min read
How do you create custom database indexes in Magento?
-
- 1 min read
How does Yii’s “Response” class work?
-
- 1 min read
What are the key publications and blogs focused on Ghost?
-
- 1 min read
Explain how FuelPHP supports pagination.
-
- 1 min read
How do you submit a package to the Concrete marketplace?
-
- 1 min read
How do you authenticate API requests in Magento?
-
- 1 min read
What is schema markup and how can you implement it in WordPress?
-
- 1 min read
Explain how to use Ghost theme helpers and tags.
-
- 1 min read
How does FuelPHP’s view layer differ from other PHP frameworks?
-
- 1 min read
How do you secure Joomla’s email forms from spam?
-
- 1 min read
What is the `ShouldQueue` interface in Laravel?
-
- 1 min read
How do you manage and optimize Ghost’s static assets?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework