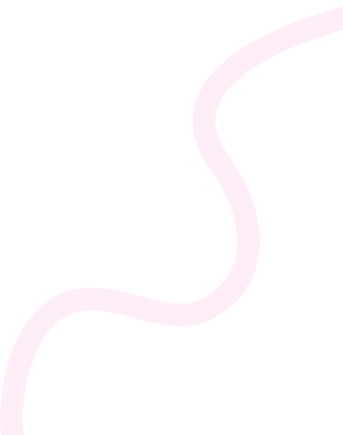
- Home
- 199 Zend Framework Interview Questions and Answers 2024
- How do you create and use a custom Zend_Db_Adapter?
How do you create and use a custom Zend_Db_Adapter?
Creating and using a custom Zend_Db_Adapter
in Zend Framework allows you to extend or modify database functionality to suit your needs. Here’s how you can create and use a custom Zend_Db_Adapter
:
1. Create the Custom Adapter Class
You need to create a new class that extends Zend_Db_Adapter_Abstract
or one of the existing adapters like Zend_Db_Adapter_Pdo_Mysql
, depending on your requirements.
Example
<?php
class My_Custom_Db_Adapter extends Zend_Db_Adapter_Pdo_Mysql
{
// Override or extend any methods here
// Example: Custom query logging
public function query($sql, $bind = array())
{
// Log the query (or do anything custom)
error_log("Executing query: " . $sql);
// Call parent method to keep the default behavior
return parent::query($sql, $bind);
}
}
?>
In this example, the custom adapter extends the MySQL adapter (Zend_Db_Adapter_Pdo_Mysql
) and overrides the query()
method to log queries.
2. Register Your Custom Adapter
To use this custom adapter in your application, you need to register it with Zend Framework. You can do this in your application.ini
configuration file or during runtime.
Option 1: Using application.ini
You can specify the adapter class directly in your application.ini
configuration:
Example
<?php
resources.db.adapter = "My_Custom_Db_Adapter"
resources.db.params.host = "localhost"
resources.db.params.username = "root"
resources.db.params.password = "password"
resources.db.params.dbname = "my_database"
?>
Option 2: During Runtime Alternatively, you can instantiate the custom adapter programmatically:
Example
<?php
// Use custom adapter during runtime
$config = array(
'host' => 'localhost',
'username' => 'root',
'password' => 'password',
'dbname' => 'my_database',
);
$dbAdapter = new My_Custom_Db_Adapter($config);
?>
3. Use the Custom Adapter
Once the adapter is registered, you can use it as you would any Zend_Db_Adapter
. For example:
Example
<?php
// Perform a query using the custom adapter
$dbAdapter->query('SELECT * FROM users');
?>
If you’re using Zend’s resources management, you can retrieve the adapter from the bootstrap:
Example
<?php
$dbAdapter = Zend_Registry::get('db');
?>
4. Extending Functionality
You can customize your adapter to fit your needs, such as:
- Query logging
- Custom connection handling
- Adding profiling or caching layers
- Handling specific database behavior
Example: Custom Adapter with Connection Pooling
You could add functionality like connection pooling or load balancing by modifying how the adapter connects to the database:
Example
<?php
class My_Custom_Db_Adapter extends Zend_Db_Adapter_Pdo_Mysql
{
protected $_pool = array();
public function getConnection()
{
if (empty($this->_pool)) {
// Initialize a new connection pool
for ($i = 0; $i < 5; $i++) {
$this->_pool[] = parent::getConnection();
}
}
// Return a connection from the pool
return array_pop($this->_pool);
}
public function closeConnection()
{
// Return the connection back to the pool
$this->_pool[] = $this->_connection;
$this->_connection = null;
}
}
?>
Related Questions & Topics
-
- 1 min read
Describe the process of integrating Slim Framework with a serverless architecture.
-
- 1 min read
Can you describe the process of integrating a CMS with marketing automation tools?
-
- 1 min read
Describe the role of Zend_Db_Adapter_Abstract.
-
- 1 min read
Explain how migrations work in FuelPHP.
-
- 1 min read
What is Zend_Http_Response and how is it used?
-
- 1 min read
What are some common performance issues with WordPress sites?
-
- 1 min read
Explain Phalcon’s support for multi-language applications.
-
- 1 min read
What is the PrestaShop Cart Rule system and how does it work?
-
- 1 min read
What are the best practices for database optimization in Magento?
-
- 1 min read
How do you import data into Concrete?
-
- 1 min read
How do you add a custom form to a Concrete page?
-
- 1 min read
How do you create and use TYPO backend layouts?
-
- 1 min read
How do you handle database errors in Ghost?
-
- 1 min read
How do you integrate SilverStripe with external authentication providers?
-
- 1 min read
What is the role of Yii’s “Request” class in handling HTTP requests?
-
- 1 min read
How do you optimize TYPO performance for high traffic sites?
-
- 1 min read
How do you implement RESTful APIs in FuelPHP?
-
- 1 min read
What is the role of Phalcon’s PhalconMvcModelMetaDataMemory class?
-
- 1 min read
How does Phalcon’s query builder support pagination?
-
- 1 min read
Can you explain the role of A/B testing in a CMS?
-
- 1 min read
How do you use Phalcon’s PhalconCacheFrontend classes?
-
- 1 min read
Explain TYPO’s method for managing user-specific settings.
-
- 1 min read
How does FuelPHP’s view layer differ from other PHP frameworks?
-
- 1 min read
What are the different ways to handle form submissions in SilverStripe?
-
- 1 min read
Describe how to add custom fields to Ghost posts and pages.
-
- 1 min read
How do you configure user roles and permissions in a CMS?
-
- 1 min read
Explain how Zend_Db_Table_Select helps in querying databases.
-
- 1 min read
What is Phalcon’s PhalconMvcModelTransactionFailed class used for?
-
- 1 min read
How can you define routes in a closure in Laravel?
-
- 1 min read
What are Zend_Db_Expr and its usage?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework