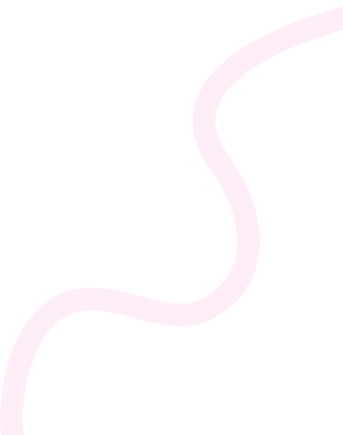
- Home
- 199 Yii Interview Questions and Answers 2024
- How do you implement a custom error handler in Yii?
How do you implement a custom error handler in Yii?
Implementing a custom error handler in Yii allows you to manage how errors and exceptions are displayed or logged in your application. Here’s a concise guide to set up a custom error handler in Yii.
Minimal Steps to Implement a Custom Error Handler in Yii
Create a Custom Error Handler Class:
- Create a new PHP file for your custom error handler (e.g.,
components/CustomErrorHandler.php
).
Example: CustomErrorHandler.php
Example
<?php
namespace app\components;
use Yii;
use yii\web\HttpException;
use yii\web\ErrorHandler;
class CustomErrorHandler extends ErrorHandler
{
protected function renderException($exception)
{
// Customize the response for different types of exceptions
if ($exception instanceof HttpException) {
Yii::$app->response->statusCode = $exception->statusCode;
return $this->renderAjaxError($exception); // Optionally, customize for AJAX
}
// Default error response
Yii::$app->response->statusCode = 500;
return $this->renderErrorPage($exception);
}
protected function renderErrorPage($exception)
{
// Render your custom error view
return $this->renderFile('@app/views/site/error.php', ['exception' => $exception]);
}
}
?>
Register the Custom Error Handler in Configuration:
- Open your
config/web.php
file and replace the default error handler with your custom one.
Example
<?php
'components' => [
'errorHandler' => [
'class' => 'app\components\CustomErrorHandler',
'errorAction' => 'site/error', // Optional: Redirect to a specific action
],
],
?>
Create an Error View:
- Create a view file (e.g.,
views/site/error.php
) to display error messages.
Example: error.php
Example
<?php
<?php
use yii\helpers\Html;
$this->title = 'Error';
?>
<div class="site-error">
<h1><?= Html::encode($this->title) ?></h1>
<div class="alert alert-danger">
<p><?= nl2br(Html::encode($exception->getMessage())) ?></p>
</div>
</div>
?>
Test Your Custom Error Handler:
- Trigger an error (e.g., by accessing a nonexistent route) to see if your custom error handling works.
Related Questions & Topics
Other Interview Question Answers
-
- 1 min read
How do you configure Yii’s “URL Manager” for SEO-friendly URLs?
-
- 1 min read
What are the steps for implementing GDPR features in PrestaShop?
-
- 1 min read
Describe the process of setting up continuous integration and deployment with Symfony.
-
- 1 min read
What are Phalcon’s best practices for managing application dependencies?
-
- 1 min read
How do you use soft deletes in Laravel?
-
- 1 min read
How do you use Xdebug with Magento?
-
- 1 min read
Explain how to handle order management in Drupal Commerce.
-
- 1 min read
Describe the process of using Slim Framework with a content management system (CMS).
-
- 1 min read
How can you implement a custom view decorator in Zend Framework?
-
- 1 min read
How do you manage blog posts in Concrete?
-
- 1 min read
How do you secure Joomla’s log files?
-
- 1 min read
Explain how to handle named route parameters in Laravel.
-
- 1 min read
How do you handle different content types and encoding formats in Slim Framework?
-
- 1 min read
How do you implement authentication in CakePHP?
-
- 1 min read
How do you handle bulk product updates in PrestaShop?
-
- 1 min read
How do you prevent XSS (Cross-Site Scripting) attacks in Joomla?
-
- 1 min read
What is the role of the “ConcreteCore” namespace in Concrete?
-
- 1 min read
Explain how Yii supports database migrations and rollbacks.
-
- 1 min read
What is RequireJS, and how does it function in Magento?
-
- 1 min read
Describe the process of attending Ghost conferences and meetups.
-
- 1 min read
What are Phalcon’s best practices for securing API endpoints?
-
- 1 min read
What is a PrestaShop override and how is it used?
-
- 1 min read
What is TYPO’s method for integrating with third-party authentication providers?
-
- 1 min read
What are some best practices for managing user access in Ghost?
-
- 1 min read
What is the use of the migration library in CodeIgniter?
-
- 1 min read
How does Phalcon’s ORM support relationships between models?
-
- 1 min read
What is the purpose of register_sidebar()?
-
- 1 min read
How does Yii compare to other PHP frameworks like Laravel or Symfony?
-
- 1 min read
Explain how to use Composer for managing Drupal dependencies.
-
- 1 min read
What are some common security vulnerabilities in Drupal?
Other Interview Question Answers
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework