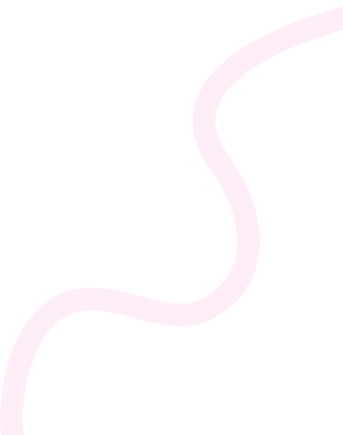
- Home
- Fuel PHP Interview Questions and Answers 2024
- How do you perform eager loading in FuelPHP ORM?
How do you perform eager loading in FuelPHP ORM?
In FuelPHP ORM, eager loading is a technique used to optimize database queries by fetching related models in a single query rather than executing multiple queries. This is done using the with()
method, which specifies the related models that should be loaded along with the main model. By leveraging eager loading, you can significantly improve performance, especially in cases where you’re dealing with large datasets and multiple relations.
Example 1: Loading Comments with Posts
Let’s say you have a blog where each post has multiple comments. Instead of querying posts first and then querying for each post’s comments separately, you can use eager loading to fetch everything in one query:
Example
<?php
$results = Model_Post::query()->with('comments')->get();
?>
In this case, the query retrieves all posts along with their associated comments in a single database query. This reduces the number of queries executed, as opposed to the “N+1 problem,” where one query is executed to get the posts and another query for each post to fetch its comments.
Example 2: Loading Author and Comments with Posts
You can also load multiple related models at once. Suppose each post has both an author and comments. You can load both relationships together:
Example
<?php
$results = Model_Post::query()->with(['author', 'comments'])->get();
?>
Here, the query retrieves all posts, their respective authors, and associated comments in one go. This can be particularly useful when you want to display comprehensive information about each post on a page, without causing a performance bottleneck due to excessive querying.
Example 3: Loading Nested Relations
FuelPHP ORM also allows you to load nested relations. For instance, if each comment on a post has replies (comments on comments), you can eagerly load this nested relation as well:
Example
<?php
$results = Model_Post::query()->with(['comments', 'comments.replies'])->get();
?>
This will retrieve all posts, their comments, and the replies to each comment. By structuring your query this way, you avoid running separate queries for posts, comments, and replies, making the process much more efficient.
Example 4: Filtering Eager Loaded Data
Sometimes, you might want to filter the related data you’re eager loading. For instance, if you want to only load comments that are marked as approved:
Example
<?php
$results = Model_Post::query()
->with(['comments' => function($query) {
$query->where('status', 'approved');
}])
->get();
?>
This query retrieves all posts and only their approved comments, helping you avoid loading unnecessary data and ensuring the query remains optimized.
Key Benefits of Eager Loading
- Performance: Fewer queries result in faster page loads and lower database load.
- Clarity: The code is easier to read and understand as all related data is fetched in a single step.
- Scalability: Eager loading prevents the “N+1” query problem, making the application more scalable when dealing with large datasets.
By using eager loading in FuelPHP ORM, you ensure that related data is efficiently retrieved, minimizing the number of database queries and improving overall application performance.
Related Questions & Topics
-
- 1 min read
How do you handle multiple databases in a CakePHP application?
-
- 1 min read
What is the role of the PrestaShop Order State system?
-
- 1 min read
How do you handle form submission and data binding?
-
- 1 min read
How do you secure Joomla’s third-party extensions?
-
- 1 min read
Explain how Slim Framework’s Callable routes work.
-
- 1 min read
How do you implement custom user profiles in Joomla?
-
- 1 min read
How can you use Zend_Db_Adapter_Pdo_Mysql for MySQL databases?
-
- 1 min read
How do you consume external APIs in Drupal?
-
- 1 min read
How do you install Joomla on a web server?
-
- 1 min read
What is the process for migrating content from one CMS to another?
-
- 1 min read
How do you implement real-time notifications in FuelPHP?
-
- 1 min read
What is the role of Yii’s “Request” class in handling HTTP requests?
-
- 1 min read
What are Phalcon’s features for handling XML data?
-
- 1 min read
How do you handle TYPO file uploads and management?
-
- 1 min read
What is a bake command in CakePHP?
-
- 1 min read
Explain the usage of ErrorHandler in Slim Framework.
-
- 1 min read
What is a REST controller, and how does FuelPHP support it?
-
- 1 min read
Explain the concept of site and network admins in Multisite.
-
- 1 min read
What is Zend_Translate and how is it used for localization?
-
- 1 min read
Describe the steps to integrate Slim Framework with a monitoring tool.
-
- 1 min read
What are PHPUnit tests, and how do you integrate them in FuelPHP?
-
- 1 min read
How does FuelPHP handle configuration settings?
-
- 1 min read
How do you create a responsive WordPress theme?
-
- 1 min read
What are Symfony’s best practices for ensuring zero-downtime deployments?
-
- 1 min read
How does Yii’s “Query Builder” facilitate database interactions?
-
- 1 min read
Describe the usage of Yii’s Formatter component.
-
- 1 min read
How do you use query caching in CodeIgniter?
-
- 1 min read
What are WordPress themes and how do they differ from plugins?
-
- 1 min read
How do you use Slim Framework with an ORM for database migrations?
-
- 1 min read
How does FuelPHP handle assets (CSS, JS) efficiently?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework