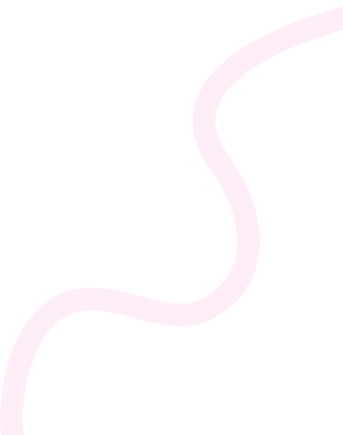
- Home
- Fuel PHP Interview Questions and Answers 2024
- How do you set up FuelPHP with Docker for development?
How do you set up FuelPHP with Docker for development?
To set up FuelPHP with Docker for development, follow these detailed steps:
1. Create a Dockerfile
The first step is to create a Dockerfile
that will define your application’s environment. In this example, we are using the official PHP image with Apache and setting up PDO for MySQL:
Example
<?php
# Use the PHP 7.4 Apache image
FROM php:7.4-apache
# Install necessary PHP extensions
RUN docker-php-ext-install pdo pdo_mysql
# Copy all files from the current directory to /var/www/html in the container
COPY . /var/www/html
# Set the working directory in the container
WORKDIR /var/www/html
?>
This Dockerfile
:
- Uses PHP 7.4 with Apache as the base image.
- Installs PDO extensions for MySQL to connect to databases.
- Copies your application code from your local machine into the container’s
/var/www/html
directory.
2. Create a Docker Compose File
Next, create a docker-compose.yml
file to orchestrate your services. This file will define the services (e.g., the web app) and how they interact.
Example
<?php
version: '3.8'
services:
app:
build: .
ports:
- "8080:80"
volumes:
- .:/var/www/html
environment:
- APACHE_DOCUMENT_ROOT=/var/www/html/public
?>
Key points in this configuration:
build: .
tells Docker Compose to build the image using the Dockerfile in the current directory.- Ports:
8080:80
maps port 80 of the container to port 8080 on your local machine. You will access the app viahttp://localhost:8080
. - Volumes: This mounts the current directory into the container, so any changes you make to your local files will immediately reflect in the container.
- APACHE_DOCUMENT_ROOT: This sets the Apache document root to the
public
folder, which is the default for FuelPHP applications.
3. Set Up Your FuelPHP Application
Now, create or clone your FuelPHP application in the same directory where the Dockerfile
and docker-compose.yml
are located. You can clone an existing FuelPHP repository or create a new one:
Example
git clone https://github.com/fuel/fuel fuelphp-app
cd fuelphp-app
Ensure the public
directory exists, as that’s where FuelPHP serves content.
4. Run Docker
Open your terminal, navigate to the project directory (where the Dockerfile
and docker-compose.yml
are), and run the following command to start your application:
Example
docker-compose up -d
-d
runs the containers in detached mode, meaning the app will run in the background.
Docker will build your image, create the container, and start your FuelPHP app.
5. Access the Application
After Docker finishes setting up, open a browser and go to:
Example
http://localhost:8080
Example Project Structure:
Here’s how your project structure will look after completing the above steps:
Example
fuelphp-app/
│
├── Dockerfile
├── docker-compose.yml
├── public/
│ └── index.php
├── fuel/
├── app/
└── other_fuelphp_files
6. Additional Configuration (Optional)
- Database Setup: If your FuelPHP app requires a database, you can add a MySQL service to your
docker-compose.yml
like this:
Example
version: '3.8'
services:
app:
build: .
ports:
- "8080:80"
volumes:
- .:/var/www/html
environment:
- APACHE_DOCUMENT_ROOT=/var/www/html/public
db:
image: mysql:5.7
volumes:
- db-data:/var/lib/mysql
environment:
MYSQL_ROOT_PASSWORD: root_password
MYSQL_DATABASE: fuelphp_db
MYSQL_USER: fuelphp_user
MYSQL_PASSWORD: user_password
volumes:
db-data:
FuelPHP Configuration: Update fuel/app/config/development/db.php
with your database credentials:
Example
return array(
'default' => array(
'type' => 'mysql',
'connection' => array(
'hostname' => 'db',
'database' => 'fuelphp_db',
'username' => 'fuelphp_user',
'password' => 'user_password',
),
),
);
Related Questions & Topics
-
- 1 min read
Explain the TYPO TypoScript Object Browser.
-
- 1 min read
What are Phalcon’s features for working with JSON data?
-
- 1 min read
How do you perform security testing in Magento?
-
- 1 min read
Explain how to use the Views module in Drupal.
-
- 1 min read
Describe the process of creating a new module in Yii.
-
- 1 min read
How do you use Zend_Http_Client for making HTTP requests?
-
- 1 min read
How do you use Slim Framework for developing RESTful APIs with versioning?
-
- 1 min read
How do you handle route caching in Laravel?
-
- 1 min read
What is the wp_terms table and how is it used?
-
- 1 min read
Explain the role of Ghost’s static file handling in performance.
-
- 1 min read
What is the purpose of the AuthComponent::allow() method?
-
- 1 min read
How do you restrict access to content based on user roles in Drupal?
-
- 1 min read
Explain how to implement custom post type archives.
-
- 1 min read
How do you handle errors in CodeIgniter?
-
- 1 min read
What is a partial in FuelPHP views, and how do you implement it?
-
- 1 min read
How do you manage and mitigate risks during a CMS migration?
-
- 1 min read
How does FuelPHP handle caching?
-
- 1 min read
Explain how to use Joomla’s language files for localization.
-
- 1 min read
How do you implement a Joomla site with a multilingual chatbot?
-
- 1 min read
How do you handle database transactions in CakePHP?
-
- 1 min read
How do you use Zend_Db_Adapter_Pdo_Sqlsrv for SQL Server databases?
-
- 1 min read
How do you use Xdebug with Magento?
-
- 1 min read
What is the purpose of Symfony’s Profiler?
-
- 1 min read
How can you create custom product types in WooCommerce?
-
- 1 min read
What are Phalcon’s tools for managing application performance?
-
- 1 min read
What is a model in CodeIgniter?
-
- 1 min read
Explain how to implement Yii’s IdentityInterface for user authentication.
-
- 1 min read
What is CakePHP?
-
- 1 min read
How do you create a new article in Joomla?
-
- 1 min read
How do you establish relationships between models in FuelPHP ORM?
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework