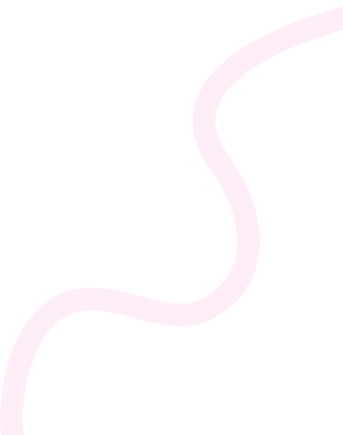
- Home
- 199 Zend Framework Interview Questions and Answers 2024
- What is Zend_Cache and how is it configured?
What is Zend_Cache and how is it configured?
Zend_Cache
is a component of the Zend Framework (now Laminas) that provides a unified API for caching data and objects. It allows developers to improve application performance by storing frequently accessed data in a cache, reducing the need for expensive database queries or computation. This can lead to faster response times and decreased server load.
Overview of Zend_Cache
- Purpose:
Zend_Cache
is used to cache data to enhance performance and reduce the workload on resources such as databases and APIs. - Supported Backends:
Zend_Cache
supports various caching backends, including:- File-based caching
- Memory-based caching (like APC, Memcached, Redis)
- Database caching
- Caching Types: You can cache different types of data, such as:
- Data cache (e.g., arrays, strings)
- Page cache (for entire page responses)
- Object cache (for objects or resources)
Configuring Zend_Cache
Include the Zend_Cache Component:
- Make sure you have the Zend Framework installed and properly configured in your project.
Create a Cache Configuration:
- Define your caching options in a configuration file or array. You can specify the backend type, options, and other caching settings.
Example: Configuration Array
Example
<?php
$cacheConfig = [
'backend' => 'File',
'frontend' => [
'name' => 'Core',
'options' => [
'lifetime' => 7200, // Cache lifetime in seconds
'automatic_serialization' => true,
],
],
'backendOptions' => [
'cache_dir' => '/path/to/cache/', // Directory to store cache files
],
];
?>
Initialize the Cache:
- Create an instance of
Zend_Cache_Manager
orZend_Cache
and pass the configuration to it.
Example: Initializing Cache
Example
<?php
use Zend_Cache;
use Zend_Cache_Manager;
// Create cache instance
$cache = Zend_Cache::factory(
'Core', // frontend name
'File', // backend name
['lifetime' => 7200, 'automatic_serialization' => true],
['cache_dir' => '/path/to/cache/']
);
?>
Using the Cache:
- You can now use the cache instance to store, retrieve, and delete cached data.
Example: Storing and Retrieving Cache
Example
<?php
// Store data in cache
$cache->save($data, 'myCacheKey');
// Retrieve data from cache
$cachedData = $cache->load('myCacheKey');
if ($cachedData === false) {
// Cache miss; retrieve data from the source
$cachedData = getDataFromSource();
$cache->save($cachedData, 'myCacheKey');
}
?>
Clearing the Cache:
- You can clear specific cached items or clear the entire cache as needed.
Example: Clearing Cache
Example
<?php
// Clear a specific cache entry
$cache->remove('myCacheKey');
// Clear all cache entries
$cache->clean(Zend_Cache::CLEANING_MODE_ALL);
?>
Related Questions & Topics
Other Interview Question Answers
-
- 1 min read
How do you implement custom form validation rules in SilverStripe?
-
- 1 min read
What are nodes in Drupal?
-
- 1 min read
How do you deploy a Ghost site to production?
-
- 1 min read
How do you use Joomla’s Media Manager API?
-
- 1 min read
How do you enforce SSL in CodeIgniter?
-
- 1 min read
Explain the role of Ghost’s static file handling in performance.
-
- 1 min read
What is the purpose of the setup:upgrade command in Magento?
-
- 1 min read
How do you handle TYPO user groups and access control lists (ACLs)?
-
- 1 min read
What is the role of the TYPO File Abstraction Layer (FAL)?
-
- 1 min read
What are the main components of a FuelPHP application?
-
- 1 min read
How do you integrate SilverStripe with external APIs and services?
-
- 1 min read
Can you describe the process of creating and managing custom fields in a CMS?
-
- 1 min read
Describe the purpose of Twig template inheritance.
-
- 1 min read
How do you create a FuelPHP package using the oil command?
-
- 1 min read
How does Doctrine handle relationships between entities?
-
- 1 min read
How do you handle form submissions and validation in SilverStripe?
-
- 1 min read
How do you handle custom data import and export functionality in SilverStripe?
-
- 1 min read
How do you create and use a custom Zend_Db_Adapter?
-
- 1 min read
How do you handle database migrations in Phalcon?
-
- 1 min read
How can you customize a block in Concrete?
-
- 1 min read
Describe the process of translating content dynamically in Symfony.
-
- 1 min read
How do you implement custom error handling in SilverStripe?
-
- 1 min read
How do you create and use custom SilverStripe modules?
-
- 1 min read
How do you configure the database settings in FuelPHP?
-
- 1 min read
What are Yii’s “Event Handlers” and how are they used?
-
- 1 min read
How do you install and configure a new module in PrestaShop?
-
- 1 min read
How do you connect to multiple databases in CodeIgniter?
-
- 1 min read
How does Symfony manage routing?
-
- 1 min read
How do you override core Joomla functions?
-
- 1 min read
What is the role of service tags in Symfony?
Other Interview Question Answers
-
- 1 min read
AI and Data Scientist
-
- 1 min read
Android
-
- 1 min read
Angular
-
- 1 min read
API Design
-
- 1 min read
ASP.NET Core
-
- 1 min read
AWS
-
- 1 min read
Blockchain
-
- 1 min read
C++
-
- 1 min read
CakePHP
-
- 1 min read
Code Review
-
- 1 min read
CodeIgniter
-
- 1 min read
Concrete5
-
- 1 min read
Cyber Security
-
- 1 min read
Data Analyst
-
- 1 min read
Data Structures & Algorithms
-
- 1 min read
Design and Architecture
-
- 1 min read
Design System
-
- 1 min read
DevOps
-
- 1 min read
Docker
-
- 1 min read
Drupal
-
- 1 min read
Flutter
-
- 1 min read
FuelPHP
-
- 1 min read
Full Stack
-
- 1 min read
Game Developer
-
- 1 min read
Ghost
-
- 1 min read
Git and GitHub
-
- 1 min read
Go Roadmap
-
- 1 min read
GraphQL
-
- 1 min read
HTML
-
- 1 min read
Java
-
- 1 min read
JavaScript
-
- 1 min read
Joomla
-
- 1 min read
jquery
-
- 1 min read
Kubernetes
-
- 1 min read
Laravel
-
- 1 min read
Linux
-
- 1 min read
Magento
-
- 1 min read
MLOps
-
- 1 min read
MongoDB
-
- 1 min read
MySql
-
- 1 min read
Node.js
-
- 1 min read
October CMS
-
- 1 min read
Phalcon
-
- 1 min read
PostgreSQL
-
- 1 min read
PrestaShop
-
- 1 min read
Product Manager
-
- 1 min read
Prompt Engineering
-
- 1 min read
Python
-
- 1 min read
QA
-
- 1 min read
React
-
- 1 min read
React Native
-
- 1 min read
Rust
-
- 1 min read
SilverStripe
-
- 1 min read
Slim
-
- 1 min read
Software Architect
-
- 1 min read
Spring Boot
-
- 1 min read
SQL
-
- 1 min read
Symfony
-
- 1 min read
System Design
-
- 1 min read
Technical Writer
-
- 1 min read
Terraform
-
- 1 min read
TypeScript
-
- 1 min read
TYPO3
-
- 1 min read
UX Design
-
- 1 min read
Vue
-
- 1 min read
WordPress
-
- 1 min read
xml
-
- 1 min read
Yii
-
- 1 min read
Zend Framework